GitHub Actions Workflow Strategies: Parallel vs. Dependent Workflows
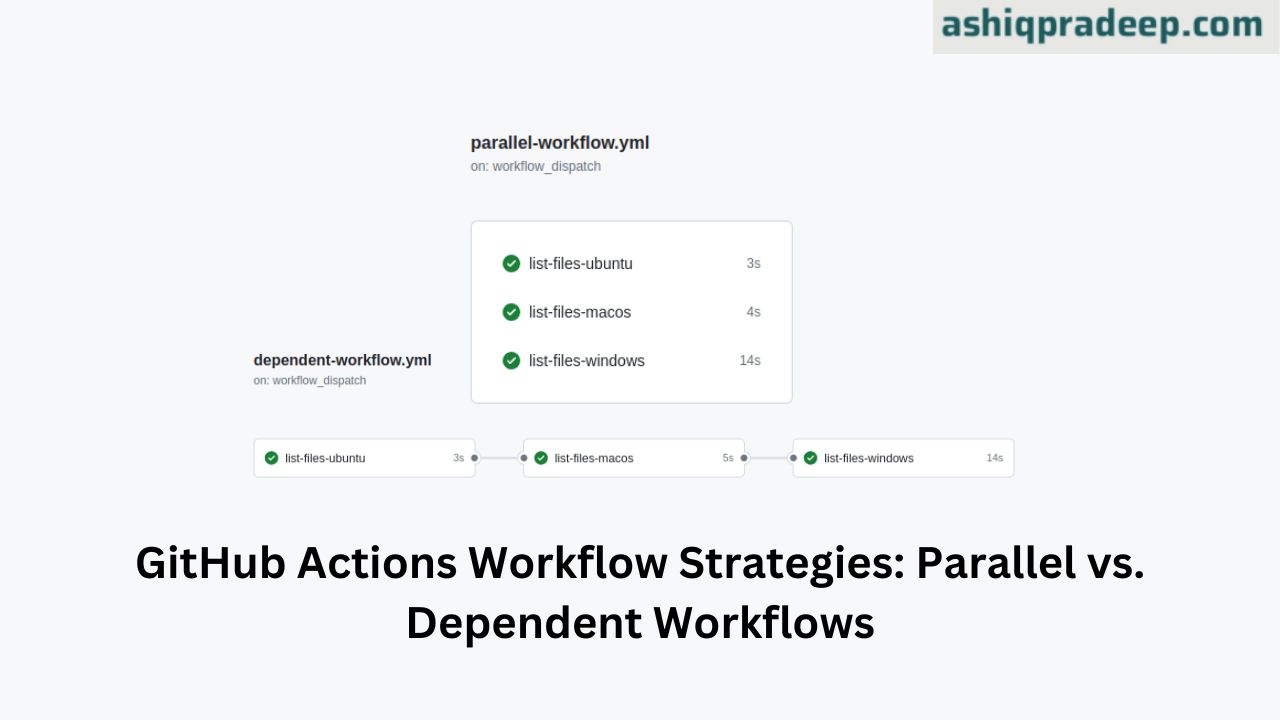
When designing GitHub Actions workflows, understanding the difference between parallel and dependent workflows is crucial for optimizing your CI/CD processes. Here's a straightforward look at both strategies, their benefits, drawbacks, and best-use cases to help you decide which approach suits your project needs.
Parallel Workflows
Definition:
Parallel workflows run multiple jobs simultaneously, with each job operating independently. This allows tasks to execute concurrently, reducing overall build time.
Benefits:
Speed: Significantly reduces the total time required for workflows to complete by running jobs in parallel.
Efficiency: Maximizes resource utilization by ensuring all available computational power is used effectively.
Drawbacks:
Resource Intensive: Running multiple jobs at the same time can consume a lot of resources.
Complexity: Managing dependencies and coordinating outputs from parallel jobs can introduce complexity.
Best-Use Cases:
Testing: Running different test suites (e.g., unit tests and integration tests) simultaneously.
Multi-Platform Builds: Building and testing your project across multiple operating systems at the same time.
Example:
name: Parallel Workflow Example
on: [workflow_dispatch]
jobs:
list-files-ubuntu:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: List files on Ubuntu
run: ls -al
list-files-macos:
runs-on: macos-latest
steps:
- uses: actions/checkout@v2
- name: List files on macOS
run: ls -al
list-files-windows:
runs-on: windows-latest
steps:
- uses: actions/checkout@v2
- name: List files on Windows
run: Get-ChildItem -Path . -Recurse
Explanation:
In this example, three jobs (list-files-ubuntu, list-files-macos, and list-files-windows) run in parallel. Each job runs independently on a different operating system (Ubuntu, macOS, Windows) and lists files in the directory. The jobs do not depend on each other and start simultaneously when a push event triggers the workflow.
Here is an example of a workflow result:
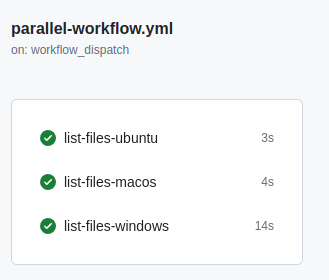
Dependent Workflows
Definition:
Dependent workflows run jobs sequentially, where one job depends on the successful completion of another. This creates a chain of tasks that follow a specific order.
Benefits:
Reliability: Ensures that each step is only executed if the previous step has completed successfully, reducing the risk of errors propagating through the workflow.
Simplicity: Easier to manage and understand as the order of execution is clearly defined.
Drawbacks:
Time-Consuming: Sequential execution can be slower as each job waits for its predecessor to complete.
Bottlenecks: If one step takes significantly longer than others, it can delay the entire workflow.
Best-Use Cases:
Build Pipelines: When building and deploying an application, where each step (build, test, deploy) depends on the success of the previous one.
Data Processing: When processing data in stages, ensuring that each stage's output is correct before moving to the next.
Example:
name: Dependent Workflow Example
on: [workflow_dispatch]
jobs:
list-files-ubuntu:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: List files on Ubuntu
run: ls -al
list-files-macos:
runs-on: macos-latest
needs: list-files-ubuntu
steps:
- uses: actions/checkout@v2
- name: List files on macOS
run: ls -al
list-files-windows:
runs-on: windows-latest
needs: list-files-macos
steps:
- uses: actions/checkout@v2
- name: List files on Windows
run: Get-ChildItem -Path . -Recurse
Explanation:
In this example, the jobs run sequentially, creating a dependent workflow. The list-files-macos job runs only after the list-files-ubuntu job completes successfully. Similarly, the list-files-windows job runs only after the list-files-macos job completes. The needs keyword establishes these dependencies, ensuring that jobs run in the specified order.
Here is an example of a workflow result:

Choosing the Right Strategy
Deciding between parallel and dependent workflows depends on the nature of your tasks and project requirements. Here are some guidelines to help you choose:
Use Parallel Workflows If:
You need to speed up execution time by running tasks concurrently.
Your tasks are independent of each other.
You have ample resources to handle multiple simultaneous jobs.
Use Dependent Workflows If:
Tasks need to be executed in a specific order.
You require each step to succeed before moving to the next.
You need to ensure the correctness and reliability of each stage before proceeding.
By understanding the strengths of both parallel and dependent workflows, you can create efficient, reliable, and scalable CI/CD pipelines in GitHub Actions.