How to Create a Reusable Workflow in GitHub Actions Using Inputs
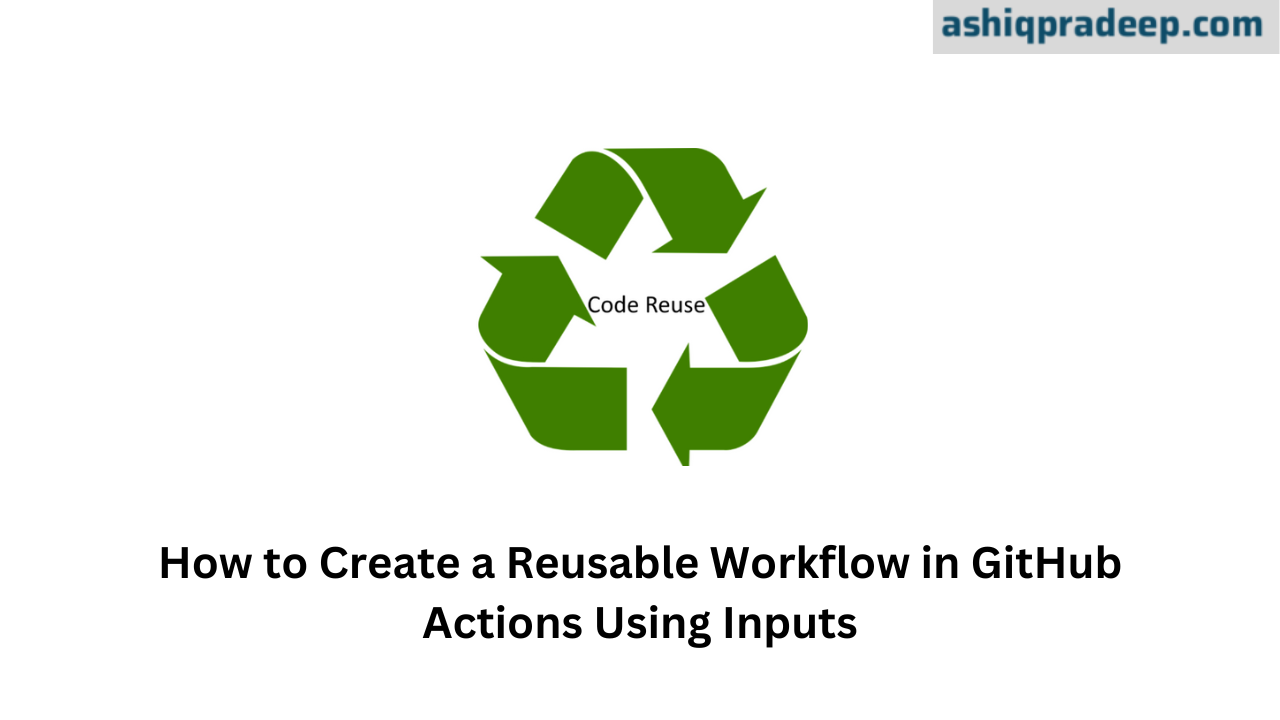
GitHub Actions is a versatile tool for automating your software development workflows. One of its most powerful features is the ability to create reusable workflows. Reusable workflows, combined with inputs, enable you to define a set of actions once and then reuse them across multiple workflows or repositories, making your automation processes more modular and easier to maintain.
What are Reusable Workflows?
Reusable workflows are pre-defined sets of actions that can be called from other workflows. They allow you to centralize and share common workflows, ensuring consistency and reducing duplication.
What are Inputs?
Inputs in GitHub Actions are parameters that you define within your workflows. They allow you to pass data into the workflow to control its behavior, making the workflows more flexible and reusable.
Defining a Reusable Workflow with Inputs
Reusable workflows are defined in YAML files within the .github/workflows directory of your repository. They use the workflow_call trigger to allow other workflows to invoke them. You can also define inputs to make the workflow customizable.
Example of a Reusable Workflow with Inputs:
# .github/workflows/reusable-workflow.yml
name: Reusable Workflow
on:
workflow_call:
inputs:
name:
description: 'Name of the person'
required: true
type: string
greeting:
description: 'Greeting message'
required: false
default: 'Hello'
type: string
jobs:
greet:
runs-on: ubuntu-latest
steps:
- name: Print greeting
run: echo "${{ inputs.greeting }}, ${{ inputs.name }}!"
In this example, the reusable workflow is defined with two inputs: name and greeting. The job greet prints a greeting message using these inputs.
Calling a Reusable Workflow
To call a reusable workflow from another workflow, use the uses keyword and specify the path to the reusable workflow file.
Example of Calling a Reusable Workflow:
# .github/workflows/caller-workflow.yml
name: Caller Workflow
on:
workflow_dispatch:
jobs:
call-reusable-workflow:
uses: ./.github/workflows/reusable-workflow.yml
with:
name: 'Pradeep Kudukkil'
greeting: 'Welcome'
In this example, the caller-workflow.yml file calls the reusable workflow defined in reusable-workflow.yml, passing the inputs name and greeting.
Advanced Usage of Reusable Workflows with Inputs
Default Values:
You can provide default values for inputs in reusable workflows, which will be used if no other value is provided.
inputs:
greeting:
description: 'Greeting message'
required: false
default: 'Hello'
type: string
Type Validation:
Inputs can have specific types such as string, boolean, or number, ensuring the input provided meets the expected format.
inputs:
age:
description: 'Age of the person'
required: true
type: number
Output Parameters:
Reusable workflows can also define outputs, allowing them to return data to the caller workflow.
jobs:
greet:
runs-on: ubuntu-latest
outputs:
message: ${{ steps.greet-message.outputs.result }}
steps:
- id: greet-message
run: echo "result=${{ inputs.greeting }}, ${{ inputs.name }}!" >> $GITHUB_OUTPUT
Best Practices
Modularity:
Keep reusable workflows small and focused on specific tasks.Use inputs and outputs to make them flexible and adaptable.
Documentation:
Provide clear descriptions for inputs and outputs. Document the usage of reusable workflows in your repository’s README file or a separate documentation file.
Versioning:
Use version tags for reusable workflows to manage changes and ensure stability.
Reference specific versions when calling reusable workflows.
Here is an example of workflow result:
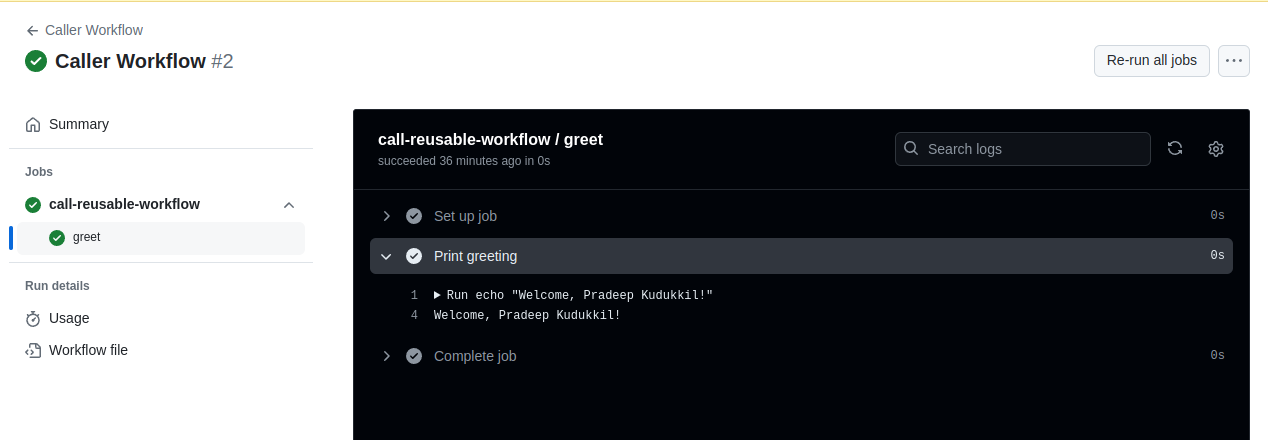
Conclusion
Creating reusable workflows in GitHub Actions using inputs provides a powerful way to standardize and streamline your automation processes. By defining and using reusable workflows with inputs, you can reduce duplication, ensure consistency, and simplify maintenance.
For more details and examples, check out My GitHub